Stack
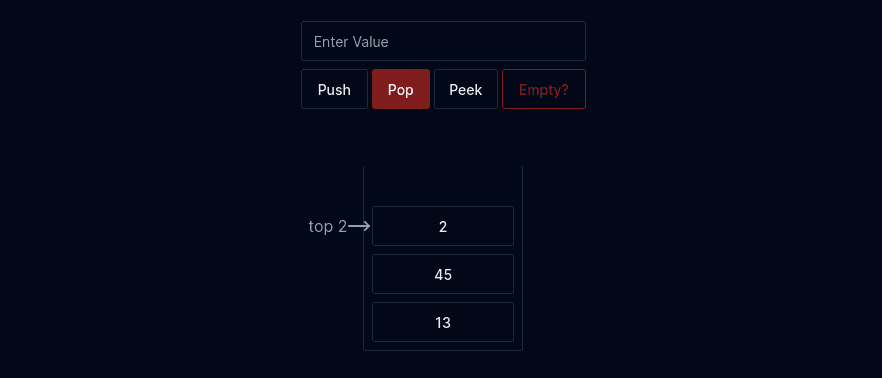
Stack
A stack is a fundamental data structure in computer science that operates on the Last In, First Out (LIFO) principle. It is commonly used for managing function calls, handling expressions, and efficient memory management.
Linked List

Linked List Introduction
A linked list is a linear data structure where elements are stored in nodes, and each node points to the next one in the sequence. Linked lists provide dynamic memory allocation and efficient insertion and deletion operations.
Binary Tree

Binary Tree Basics
A binary tree is a hierarchical data structure composed of nodes, each having at most two children. Binary trees provide a flexible and efficient way to organize and retrieve data.
Heaps

Min Heap
A Min Heap is a specialized binary heap data structure in which the value of each node is less than or equal to the values of its children, ensuring the minimum element is at the root.

Max Heap
A Max Heap is a binary heap where the value of each node is greater than or equal to the values of its children, ensuring the maximum element is at the root.
Puzzles

Tower of Hanoi Overview
The Tower of Hanoi is a classic mathematical puzzle consisting of three rods and a number of disks of different sizes. The objective is to move the entire stack of disks from one rod to another following specific rules.
Searching

Binary Search
Binary Search is a highly efficient algorithm for finding a specific target value within a sorted array or list. It works by repeatedly dividing the search range in half until the target is found.
Sorting

Bubble Sort
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. It's known for its simplicity but is less efficient for large datasets.

Selection Sort
Selection Sort is a basic sorting algorithm that divides the input list into two parts: a sorted and an unsorted region. It repeatedly selects the smallest element from the unsorted region and swaps it with the first element of the unsorted region.

Insertion Sort
Insertion Sort is a simple sorting algorithm that builds the final sorted array one element at a time. It takes each element from the unsorted part of the array and inserts it into its correct position in the already sorted part.